If you are already using Spring, then you might want to use Spring Security to secure your web resources.
To do that, we specify the URI to be secured with <security:intercept-url/>
tag:-
<beans ...>
<!-- Error pages don't need to be secured -->
<security:http pattern="/error/**" security="none"/>
<security:http auto-config="true">
<security:form-login ... />
<security:logout ... />
<security:intercept-url pattern="/top-secrets/**" access="ROLE_TOPSECRET"/>
</security:http>
...
</beans>
When users without role ROLE_TOPSECRET
access /top-secrets/kfc-secret
, they will see this default error page:-

This proves that Spring Security is doing its job. However, the default error page looks rather F.U.G.L.Y. Further, the error page may reveal too much information about the application server. The above error page shows the application runs on Jetty. If I’m a motherhacker, I would research all the possible vulnerabilities on this particular application server in attempt to hack it.
A better solution is to provide a friendly error page when the user access is denied. This can be done by specifying <security:access-denied-handler/>
tag:-
<beans ...>
<!-- Error pages don't need to be secured -->
<security:http pattern="/error/**" security="none"/>
<security:http auto-config="true">
<security:form-login ... />
<security:logout ... />
<security:access-denied-handler error-page="/error/access-denied"/>
<security:intercept-url pattern="/top-secrets/**" access="ROLE_TOPSECRET"/>
</security:http>
...
</beans>
Then, we create a simple error controller that returns the error page:-
@Controller
@RequestMapping(value = "/error")
public class ErrorController {
@RequestMapping(value = "/access-denied", method = RequestMethod.GET)
public String accessDenied() {
return "error-access-denied";
}
}
Now, the user will see this custom error page:-
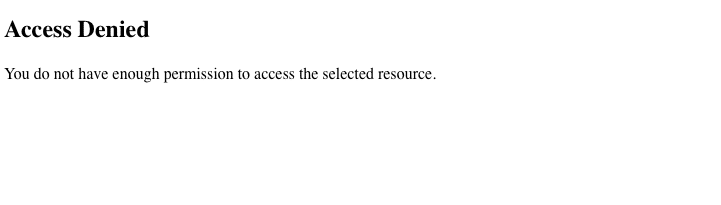
This solution is better than the previous one. However, SiteMesh doesn’t have the opportunity to decorate this error page before it gets rendered.
To fix this, we can create a simple redirect to allow the request to make a full-round trip to the server so that SiteMesh can decorate the error page:-
@Controller
@RequestMapping(value = "/error")
public class ErrorController {
@RequestMapping(value = "/router", method = RequestMethod.GET)
public String errorRouter(@RequestParam("q") String resource) {
return "redirect:/error/" + resource;
}</code>
<code>
</code>
<code> @RequestMapping(value = "/access-denied", method = RequestMethod.GET)
public String accessDenied() {
return "error-access-denied";
}
}
Then, we tweak the Spring Security to use the error router URI:-
<beans ...>
<!-- Error pages don't need to be secured -->
<security:http pattern="/error/**" security="none"/></code>
<code>
<security:http auto-config="true">
<security:form-login ... />
<security:logout ... />
<security:access-denied-handler error-page="/error/router?q=access-denied"/>
<security:intercept-url pattern="/top-secrets/**" access="ROLE_TOPSECRET"/>
</security:http>
</code>
<code> ...
</beans>
Now, the user will see this nice beautiful error page:-
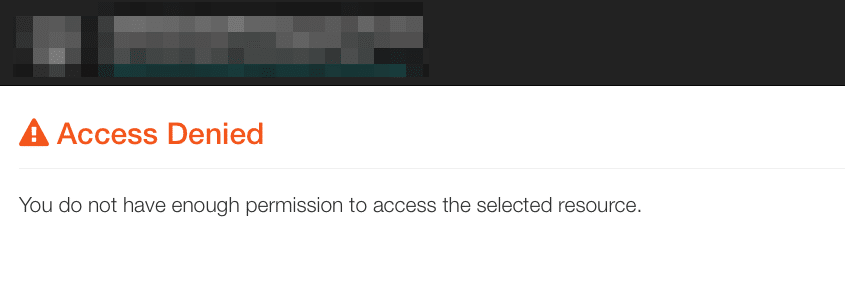
Leave a Reply