PROBLEM
Sometimes, we have very lengthy statements that look like this:-
@Service
public class LengthOfStayHeuristicServiceImpl extends HeuristicService {
@Override
public Double compute(HeuristicBean heuristicBean) {
...
setValue(map, surgeryLocationMap, LengthOfStayAlgorithm.VariableEnum.SURGERY_LOCATION_LUMBAR_OR_SACRAL_AND_LUMBOSACRAL_MINUS_CERVICAL_AND_NOT_SPECIFIED);
setValue(map, surgeryLocationMap, LengthOfStayAlgorithm.VariableEnum.SURGERY_LOCATION_LUMBAR_OR_SACRAL_MINUS_LUMBOSACRAL);
setValue(map, surgeryLocationMap, LengthOfStayAlgorithm.VariableEnum.SURGERY_LOCATION_CERVICAL_MINUS_NOT_SPECIFIED);
return new LengthOfStayAlgorithm(map).run();
}
}
When we reformat the code in IntelliJ, it becomes like this:-
@Service
public class LengthOfStayHeuristicServiceImpl extends HeuristicService {
@Override
public Double compute(HeuristicBean heuristicBean) {
...
setValue(map,
surgeryLocationMap,
LengthOfStayAlgorithm.VariableEnum.SURGERY_LOCATION_LUMBAR_OR_SACRAL_AND_LUMBOSACRAL_MINUS_CERVICAL_AND_NOT_SPECIFIED);
setValue(map,
surgeryLocationMap,
LengthOfStayAlgorithm.VariableEnum.SURGERY_LOCATION_LUMBAR_OR_SACRAL_MINUS_LUMBOSACRAL);
setValue(map,
surgeryLocationMap,
LengthOfStayAlgorithm.VariableEnum.SURGERY_LOCATION_CERVICAL_MINUS_NOT_SPECIFIED);
return new LengthOfStayAlgorithm(map).run();
}
}
There are times we really don’t want the long statements to wrap around because they look very messy.
SOLUTION
While there is no option to selectively disable just the line wrap in IntelliJ, there is a way to selectively disable code formatting.
First, we need to enable the Formatter Control.
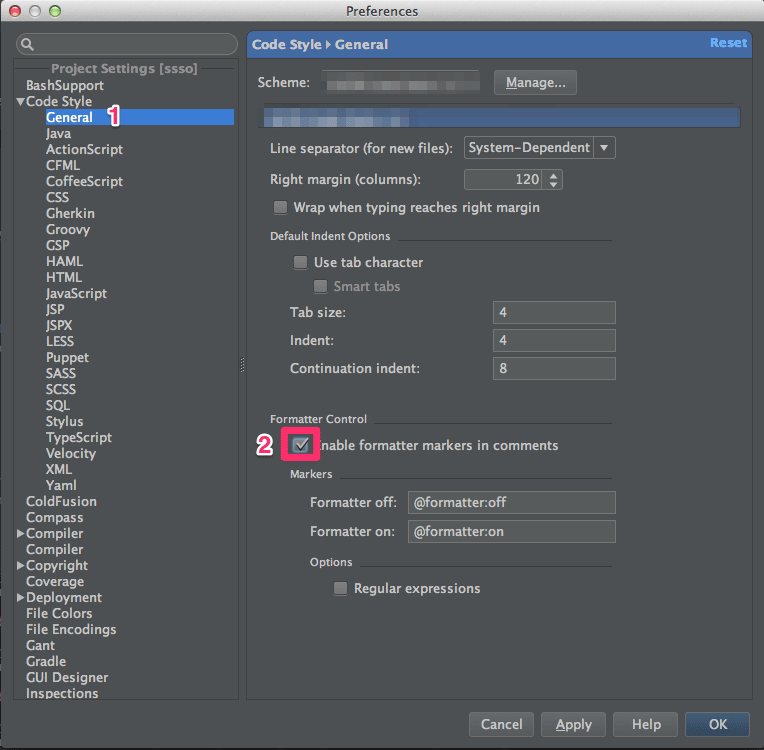
Now, we can annotate our code like this:-
@Service
public class LengthOfStayHeuristicServiceImpl extends HeuristicService {
@Override
public Double compute(HeuristicBean heuristicBean) {
...
// @formatter:off
setValue(map, surgeryLocationMap, LengthOfStayAlgorithm.VariableEnum.SURGERY_LOCATION_LUMBAR_OR_SACRAL_AND_LUMBOSACRAL_MINUS_CERVICAL_AND_NOT_SPECIFIED);
setValue(map, surgeryLocationMap, LengthOfStayAlgorithm.VariableEnum.SURGERY_LOCATION_LUMBAR_OR_SACRAL_MINUS_LUMBOSACRAL);
setValue(map, surgeryLocationMap, LengthOfStayAlgorithm.VariableEnum.SURGERY_LOCATION_CERVICAL_MINUS_NOT_SPECIFIED);
// @formatter:on
return new LengthOfStayAlgorithm(map).run();
}
}
When we reformat the code, that portion of code will remain unformatted.
Leave a Reply