PROBLEM
In order to run our web application using HTTPS, we need to enable SSL first on Jetty.
SOLUTION #1: Generating Certificate on the Fly
One clean solution by @PascalThivent is to recreate the certificate whenever Jetty starts. Not to steal any credits from him, but here’s a slightly modified configuration using more recent plugin versions:-
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>keytool-maven-plugin</artifactId>
<version>1.5</version>
<executions>
<execution>
<phase>generate-resources</phase>
<id>clean</id>
<goals>
<goal>clean</goal>
</goals>
</execution>
<execution>
<phase>generate-resources</phase>
<id>generateKeyPair</id>
<goals>
<goal>generateKeyPair</goal>
</goals>
</execution>
</executions>
<configuration>
<keystore>${project.build.directory}/jetty-ssl.keystore</keystore>
<dname>cn=localhost</dname>
<!--Both `keypass` and `storepass` must be at least 6 characters long. -->
<keypass>jetty8</keypass>
<storepass>jetty8</storepass>
<alias>jetty8</alias>
<keyalg>RSA</keyalg>
</configuration>
</plugin>
<plugin>
<groupId>org.mortbay.jetty</groupId>
<artifactId>jetty-maven-plugin</artifactId>
<version>8.1.14.v20131031</version>
...
<configuration>
<connectors>
<connector implementation="org.eclipse.jetty.server.bio.SocketConnector">
<port>7777</port>
</connector>
<connector implementation="org.eclipse.jetty.server.ssl.SslSocketConnector">
<port>7443</port>
<keystore>${project.build.directory}/jetty-ssl.keystore</keystore>
<keyPassword>jetty8</keyPassword>
<password>jetty8</password>
</connector>
</connectors>
</configuration>
</plugin>
In this case, the generated certificate is stored under the project’s build directory (“target” directory) every time we run mvn clean jetty:run. This is a great solution because it allows me to easily pass my project to my peers without the need to worry about the generated certificate.
However, a downside to this approach is because the certificate is regenerated again and again, my browser keeps prompting me to add an exception whenever I refresh the web link.
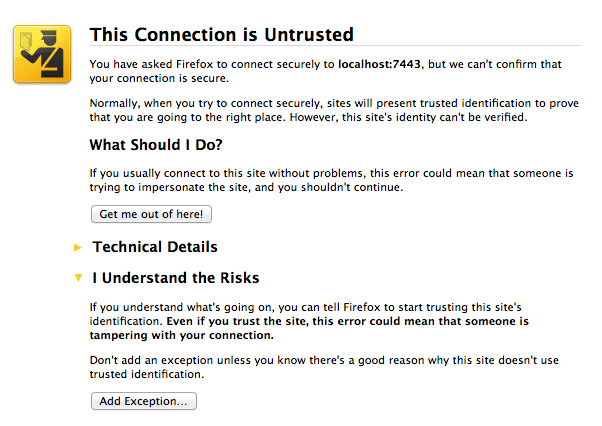
… and after adding the exception for like 5 times, it begins to get very annoying.
SOLUTION #2: Generating Certificate Once and Reuse it
Instead of regenerating the certificate again and again, I decided to generate the certificate that will last for 99999 days and store it under src/main/config directory.
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>keytool-maven-plugin</artifactId>
<version>1.5</version>
<configuration>
<keystore>${project.basedir}/src/main/config/jetty-ssl.keystore</keystore>
<dname>cn=localhost</dname>
<!--Both `keypass` and `storepass` must be at least 6 characters long. -->
<keypass>jetty8</keypass>
<storepass>jetty8</storepass>
<alias>jetty8</alias>
<keyalg>RSA</keyalg>
<validity>99999</validity>
</configuration>
</plugin>
<plugin>
<groupId>org.mortbay.jetty</groupId>
<artifactId>jetty-maven-plugin</artifactId>
<version>8.1.14.v20131031</version>
...
<configuration>
<connectors>
<connector implementation="org.eclipse.jetty.server.bio.SocketConnector">
<port>7777</port>
</connector>
<connector implementation="org.eclipse.jetty.server.ssl.SslSocketConnector">
<port>7443</port>
<keystore>${project.basedir}/src/main/config/jetty-ssl.keystore</keystore>
<keyPassword>jetty8</keyPassword>
<password>jetty8</password>
</connector>
</connectors>
</configuration>
</plugin>
To generate (or regenerate) the certificate, run mvn keytool:clean keytool:generateKeyPair.
With this approach, I just need to add an exception ONCE in my browser regardless of the number of times I restart Jetty… or when I’m still actively working on this project after 99999 days.
Leave a Reply